First steps to my RPi weather station
After my fist Pi experiment I finally decided what my final project will be: a weather station.
After the temperature I thought humidity and atmospheric pressure will be the next task:
To do that I needed two new sensors: DHT11 (don’t waste your money like I did on DHT11 buy DHT22) and BMP180.
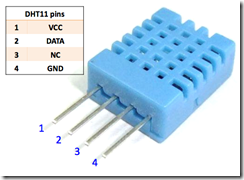
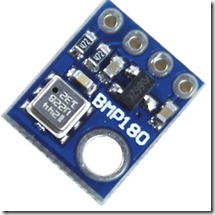
In order to get the sensor working there is no better place then the Adafruit (thanks be to god!) website, I found there all I needed to get my reading:
- https://learn.adafruit.com/using-the-bmp085-with-raspberry-pi/overview
- https://learn.adafruit.com/dht-humidity-sensing-on-raspberry-pi-with-gdocs-logging/overview
This is how I connected the two sensors:
- DHT11 gnd pin6
- DHT11 vcc pin1
- DHT11 data pin 7(gpio 4)
- BMP180 gnd pin9
- BMP180 vcc pin17
- BMP180 scl pin 5 (gpio 3 scl)
- BMP180 sda pin3 (gpio 2 sda)
I then modified the monitor.py script from my previous post (first raspberry pi project):
#!/usr/bin/python import sys import Adafruit_DHT import Adafruit_BMP.BMP085 as BMP085 import sqlite3 import os import time import glob dbname='/home/pi/templog.db' # store the temperature in the database def log_temperature(temp, pitemp, humi ,batemp, press, alt, seapress): conn=sqlite3.connect(dbname) curs=conn.cursor() curs.execute("INSERT INTO temps values(datetime('now'), (%s), (%s), (%s), (%s), (%s), (%s), (%s))" % (temp,pitemp,humi,batemp, press, alt, seapress)) # commit the changes conn.commit() conn.close() # display the contents of the database def display_data(): conn=sqlite3.connect(dbname) curs=conn.cursor() for row in curs.execute("SELECT * FROM temps"): print str(row[0])+" "+str(row[1])+" "+str(row[2])+" "+str(row[3])+" "+str(row[4])+" "+str(row[5])+" "+str(row[6])+" "+str(row[7]) conn.close() def getCPUtemperature(): res = os.popen('vcgencmd measure_temp').readline() return(res.replace("temp=","").replace("'C\n","")) # main function # This is where the program starts def main(): sensor = BMP085.BMP085() humidity, temperature = Adafruit_DHT.read_retry(Adafruit_DHT.DHT11, '4') log_temperature(temperature,getCPUtemperature(),humidity,sensor.read_temperature(),sensor.read_pressure(),sensor.read_altitude(),sensor.read_sealevel_pressure()) # display_data() if __name__=="__main__": main()
I then modified the web services rest.py code:
#!/usr/bin/env python import web from web.template import render db = web.database(dbn='sqlite', db='/home/pi/templog.db') urls = ( '/home/', 'index', '/home/(.*)','get_temp', '/pi/', 'piindex', '/pi/(.*)','get_pitemp', '/humi/', 'humiindex', '/humi/(.*)','get_humi', '/pressure/', 'pressureindex', '/pressure/(.*)','get_pressure', '/altitude/', 'altitudeindex', '/altitude/(.*)','get_altitude', '/seapressure/', 'seapressureindex', '/seapressure/(.*)','get_seapressure' ) app = web.application(urls, globals()) class index: def GET(self): temps = db.query("select timestamp, IFNULL(temp, '10') as temp, IFNULL(batemp, '10') as batemp from temps where timestamp>datetime('now','-24 hours')") #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.temp)+","+str(temp.batemp)+"]" return output class get_temp: def GET(self,hours): temps = db.query("select timestamp, IFNULL(temp, '10') as temp, IFNULL(batemp, '10') as batemp from temps where timestamp>datetime('now','-%s hours')" % hours) #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.temp)+","+str(temp.batemp)+"]" return output class piindex: def GET(self): temps = db.query("select timestamp, IFNULL(pitemp, '40') as pitemp from temps where timestamp>datetime('now','-24 hours')") #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class get_pitemp: def GET(self,hours): temps = db.query("select timestamp, IFNULL(pitemp, '40') as pitemp from temps where timestamp>datetime('now','-%s hours')" % hours) #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class humiindex: def GET(self): temps = db.query("select timestamp, IFNULL(humi, '40') as pitemp from temps where timestamp>datetime('now','-24 hours')") #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class get_humi: def GET(self,hours): temps = db.query("select timestamp, IFNULL(humi, '40') as pitemp from temps where timestamp>datetime('now','-%s hours')" % hours) #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class pressureindex: def GET(self): temps = db.query("select timestamp, IFNULL(pressure, '0') as pitemp from temps where timestamp>datetime('now','-24 hours')") #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class get_pressure: def GET(self,hours): temps = db.query("select timestamp, IFNULL(pressure, '0') as pitemp from temps where timestamp>datetime('now','-%s hours')" % hours) #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class altitudeindex: def GET(self): temps = db.query("select timestamp, IFNULL(altitude, '0') as pitemp from temps where timestamp>datetime('now','-24 hours')") #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class get_altitude: def GET(self,hours): temps = db.query("select timestamp, IFNULL(altitude, '0') as pitemp from temps where timestamp>datetime('now','-%s hours')" % hours) #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class seapressureindex: def GET(self): temps = db.query("select timestamp, IFNULL(seapressure, '0') as pitemp from temps where timestamp>datetime('now','-24 hours')") #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output class get_seapressure: def GET(self,hours): temps = db.query("select timestamp, IFNULL(seapressure, '0') as pitemp from temps where timestamp>datetime('now','-%s hours')" % hours) #select('temps') output= '' for temp in temps: output += ",['"+str(temp.timestamp)+"',"+str(temp.pitemp)+"]" return output if __name__ == "__main__": app.run()
Last but not list the index.php page on my LAMP server: Temperature Report
Here is were I got so far, next step is rain detection and wind direction and speed. I will probably need to get my breadboard for the next few steps and looking forward to get my hands on a Pi A+ (better suited Pi for this project due to the low power consumption)
Bye for now.